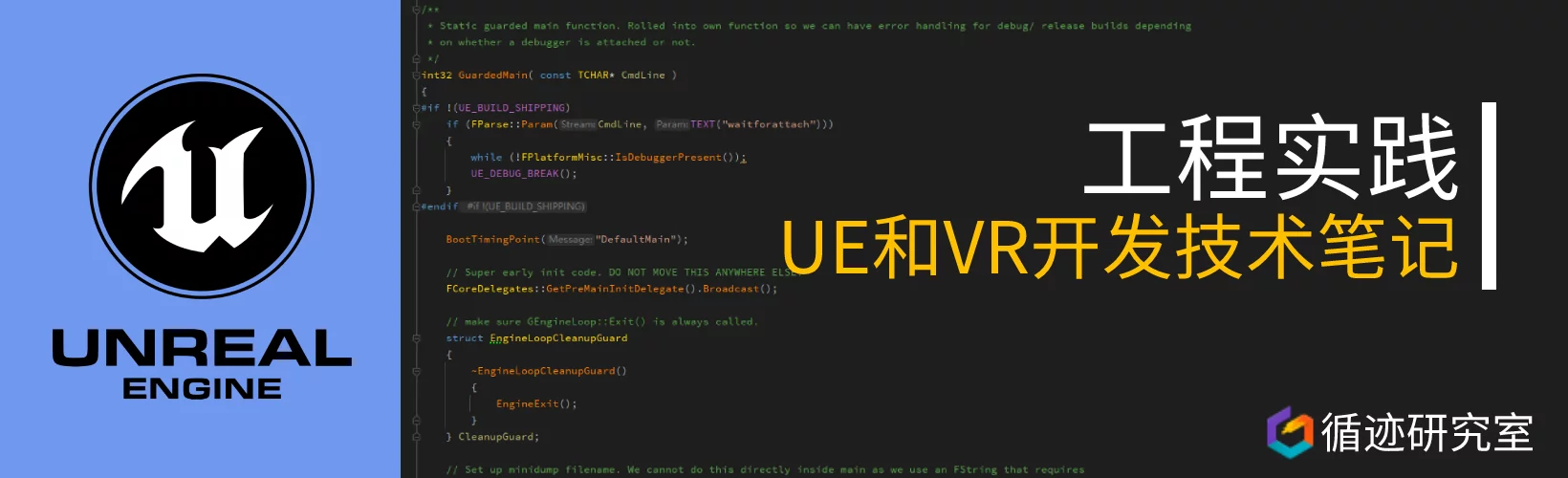
Some technical notes written in the daily cursive about UE4 and VR development, as well as some related materials. Previously scattered in imzlp.com/notes, they have been organized today, and future notes will be placed in this article.
Some technical notes written in the daily cursive about UE4 and VR development, as well as some related materials. Previously scattered in imzlp.com/notes, they have been organized today, and future notes will be placed in this article.
Because Unreal Engine destroys all objects in the current level when switching levels (OpenLevel
), we often need to retain certain objects for the next level. Today, I read some relevant code, and this article will explain how to achieve this. Unreal’s documentation does mention this (Travelling in Multiplayer), and it’s not too complicated to implement. However, the UE documentation is consistently lacking in detail, especially in Chinese, where resources are very scarce (mostly machine-translated and outdated). I couldn’t find any reliable information during my search, so I took some notes while reading the code implementation.
Recently, I have been using Protobuf in VS, and I will briefly record the process of building Protobuf using MSVC.
Due to Github Pages not supporting custom domain
HTTPS, I spent some time today setting up a reverse proxy from Nginx to Github Pages on a VPS. I used a certificate issued by Let’s Encrypt to achieve full HTTPS for the entire site (all external resource links were also changed to HTTPS). Here’s a simple record of the process.
C++ provides two logical operators ||
and &&
as well as the ,
(comma) operator in its basic syntax. We can overload these operators in a class, but we should avoid doing that. In this article, I will outline the standard descriptions and the reasons why they should not be overloaded.
In summary, because the built-in ||
and &&
have short-circuit evaluation semantics, overloading them turns them into regular function calls, yielding semantics that are entirely different from the built-in ||
and &&
. Furthermore, the ,
operator has left-to-right evaluation semantics, so if you overload it, it will also become a function call and result in semantics that differ from the built-in version.
This article mainly summarizes my insights during the learning process of template metaprogramming, and I will also include some template metaprogramming code I have written here.
In the C++14 standard (as well as C++98/11), there is a statement in Annex C Compatibility:
Change: Converting void* to a pointer-to-object type requires casting
1 | char a[10]; |
ISO C will accept this usage of pointer to void being assigned to a pointer to object type. C++ will not.
But why does operator new()
return void*
and allow assigning to T*
without an explicit conversion to T*
?
In C++, the result of a lambda-expression
is called a closure object
. This article is not intended to introduce the usage of C++ lambdas (this is covered in detail in “TC++PL” and “C++ Primer,” or you can refer to my previous summary C++11 Syntactic Sugar #lambda Expressions), but rather to analyze how lambda-expression
is implemented in Clang from the perspective of LLVM-IR.
In the previous article Breaking the Access Control Mechanism of C++ Classes, we briefly mentioned that the member access control of C++ classes (public
/protected
/private
) only limits the accessibility of member names, rather than their visibility. This article mainly analyzes the consequences of this property and how to avoid them.
It is well known that class members in C++ can have three access specifiers: public
, protected
, and private
:
[ISO/IEC 14882:2014] A member of a class can be
From the standard’s intention, it aims to hide implementation details and underlying data of a class, which is encapsulation. However, we can also bypass access restrictions through some special means.