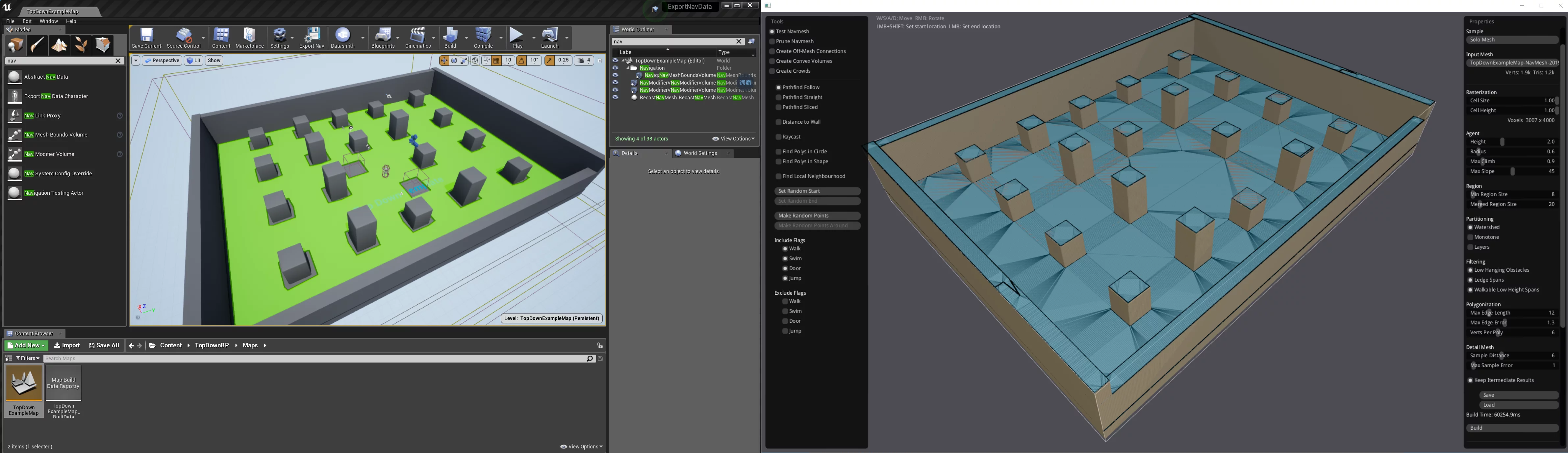
The latest version supports UE5, see the UE5.0 branch on github: ue4-export-nav-data/tree/UE5.0.
Recast Navigation is an open-source game navigation/pathfinding engine that provides pathfinding calculations for AI in games. Both UE and Unity integrate RecastNavigation to provide navigation and pathfinding calculations for games (of course, in modified versions). The UE modules NavigationSystem
and NavMesh
contain relevant code implementations. Recently, there was a requirement to export the client’s map information to a server with a non-UE network architecture, for verifying player locations on the server. It occurred to me that the navigation data generated by the client could be exported as a map of the client world, so I tinkered and wrote a UE plugin (open-sourced on Github: ue4-export-nav-data) that implements directly exporting the navigation data generated by UE. If interested, you can directly view the specific code. Based on the exported navigation data, pathfinding calculations based on Recast Navigation can be fully implemented on the server with a non-UE network architecture, seamlessly integrated with UE.
2019.12.04 Update: This plugin has been listed on the Unreal Marketplace, purchase link ExportNavigation. In support of programmer sentiment for open-source, this project’s repository on Github will not be closed, but it is unlikely to be updated. If this plugin is useful to you, feel free to purchase it on the marketplace to support the author.
Recast Navigation
First, let’s briefly introduce compiling the GitHub open-source version of Recast. The version of Recast used in the UE engine is based on this open-source version. Until UE_4.22.3
, the version of Recast used by UE is v1.4
(you can check the version information of Recast used by different engine versions from Source/Runtime/Navmesh/Recast-Readme.txt
). The source code address of RecastNavigation on GitHub is: recastnavigation. The code in recastnavigation provides navigation mesh generation and pathfinding calculation tools RecastDemo
, which can serve as an example of integrating RecastNavigation into a project.
The README.md
mentions the compilation process for various platforms. I will detail the compilation process under Windows here.
- First, download premake5 and add it to the system PATH.
- Clone the RecastNavigation code.
- Download SDL2 (choose Development Libraries) and unzip it to the
recastnavigation\RecastDemo\Contrib
directory, renaming the folder toSDL
, resulting in the following directory structure:
1 | D:\recastnavigation\RecastDemo\Contrib\SDL>tree /a |
In the
recastnavigation\RecastDemo
directory, run the commandpremake5 vs2017
(vs201x depends on the version you have installed), which will create abuild/vs2017
directory in theRecastDemo
directory containing the VS project solution.
Open
RecastDemo\Build\vs2017\recastnavigation.sln
and compile it.The compiled
RecastDemo.exe
will be located inRecastDemo\Bin
.
1 | C:\Users\imzlp\source\repos\recastnavigation\RecastDemo\Bin>tree /a /f |
Key files include: DroidSans.ttf
/RecastDemo.exe
/SDL2.dll
/Meshs/
.
Note: The obj files must be placed in the
Meshs/
directory to be recognized byRecastDemo
.
Then, you can run RecastDemo.exe
to test navigation data generation on the three default provided obj
models by clicking Build
, and saving will create a .bin
file in the directory where RecastDemo.exe
is located, which contains navigation data generated by Recast.
Note: Exporting Navmesh from UE means exporting models within the UE pathfinding range, and then using RecastDemo
to generate pathfinding data based on that model. This also results in the inability to export Navmesh effectively when adding boxes that affect pathfinding in UE during RecastDemo generation.
Plugin: ue-export-nav-data
This plugin serves as a tool for exporting RecastNavigation from UE and consists of two modules: ExportNavRuntime
and ExportNavEditor
. The editor module provides buttons in the UE editor ToolBar
for exporting navigation data.
After clicking, you can select a path, and it will generate two files: .bin
and obj
in the selected path, automatically opening the export directory in Windows Explorer.
- bin: Navigation data generated by UE, for use by external servers.
- obj: The mesh used for pathfinding in UE, which can be used to generate bin files in RecastDemo.
Additionally, I provided NavData
that can be exported at runtime, but NavMesh
is unsupported due to UE’s navigation being precomputed. Specific methods can be found in UFlibExportNavData
.
I also provided two methods in C++ and Blueprint to validate whether a position exported from the bin is a valid navigation point:
1 | bool UFlibExportNavData::IsValidNavigationPointInNavbin(const FString& InNavBinPath, const FVector& Point, const FVector InExtern = FVector::ZeroVector); |
These can be compared with the UE function UNavigationSystemV1::ProjectPointToNavigation
to verify if the exported data matches.
There’s also a method to pass a starting point to retrieve navigation path points:
1 | bool FindDetourPathByNavMesh(dtNavMesh* InNavMesh ,const FVector3& InStart, const FVector3& InEnd, std::vector<FVector3>& OutPaths); |
This can yield results consistent with UNavigationSystemV1::FindPathToLocationSynchrously
, passing world coordinates from the game, and the function internally handles transformation, returning world coordinates as well.
The advantages of this plugin include:
- It directly exports
bin
files from UE instead of exporting NavMesh’s.obj
and then usingRecastDemo
for generation. Of course, I also retained the ability to export NavMesh asobj
. - Since the navigation data is exported directly from UE, it is what you see is what you get, resolving issues where certain areas do not produce pathfinding when exporting NavMesh then generating navigation meshes in RecastDemo, and avoiding inconsistencies in pathfinding data caused by various parameters between UE and RecastDemo.
- Additionally, the ue-detour version that I’ve extracted can be seamlessly integrated into external servers, and there’s no need to convert client coordinates and server coordinates (though UE’s coordinates and Recast’s coordinates need conversion, which is already handled internally—manual conversion is unnecessary).
The conversion between UE’s coordinate system and Recast’s coordinate system can be achieved using two functions from UE4RecastHelper
:
1 | namespace UE4RecastHelper |
Library: ue-recast-detour
This is the recast detour library I extracted from UE code, located at Runtime/Navmesh/Detour
in the UE source code. The main purpose is to ensure that the UE client and the external server use consistent verification methods.
Since the version of RecastNavigation on GitHub is newer than the one used by UE, and as mentioned earlier, UE made significant modifications to Recast, I extracted the modified version used by UE to prevent discrepancies in results caused by code differences. This ensures that the client and server results are consistent.
The code is hosted on GitHub: ue4-recast-detour, with the Detour/
directory containing all the code for the Detour library. The remaining code includes the UE4RecastHelper
class that I implemented for verification and coordination transformation between UE and Recast, as well as a simple command-line program to test if world positions in UE are valid within the navigation data contained in the bin.
UE4RecastHelper
currently (2019.11.01) provides methods to serialize
/deserialize
between dtNavMesh
and bin
files, as well as a function UE4RecastHelper::dtIsValidNavigationPoint
which serves the same purpose as UNavigationSystemV1::ProjectPointToNavigation
for verifying whether a point is a valid navigation point, ensuring consistency between the UE client and the external server verification methods.
All operations supported by Detour
can be implemented on the server (as navigation data has already been retrieved from UE), and it can be expanded according to specific needs.
Lastly, a brief introduction to how to use the ue-detour.exe
tool: simply start ue4-detour.exe
from the command line to see usage instructions. First, you need to export navigation data from UE, requiring only the .bin
file, generated at, for example, D:\NavData
. Then locate ue4-detour.exe
to use the following command:
1 | D:\>ue4-detour.exe |
In Blueprint, you can also directly load the .bin
for testing:
End
The open-source repositories utilized in this article:
Update
2021.05.27 Update:
- The plugin supports UE5 and can export UE5’s NavMesh data and navigation meshes.
- Data was successfully verified using ue-detour.